Create own method in mongoose model
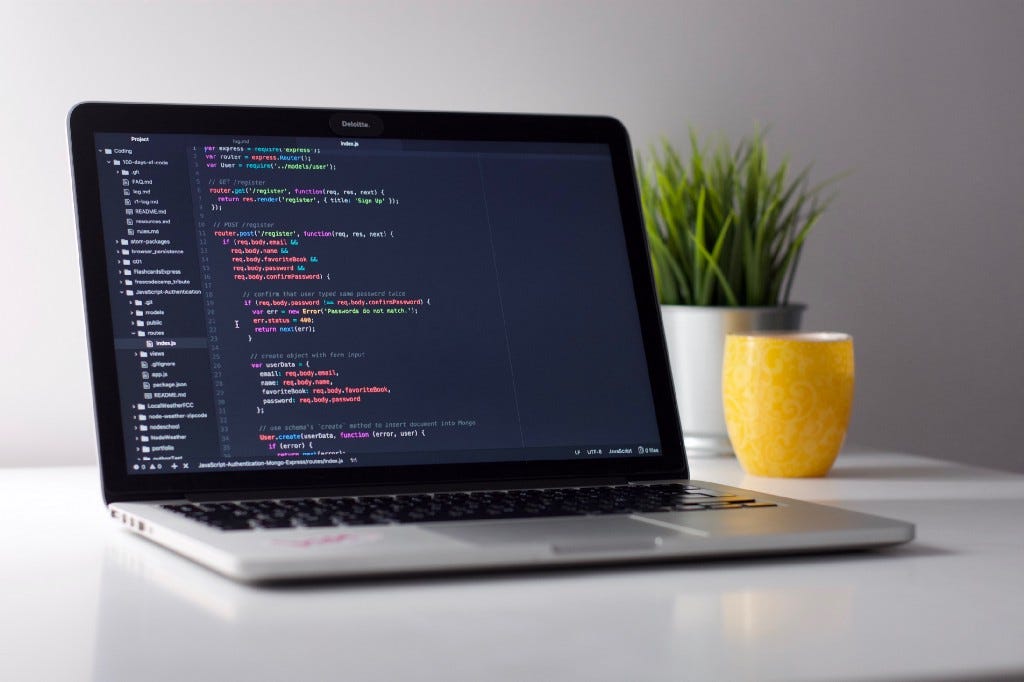
If you are familiar with the MVC design patterns, then you probably know the article from the heading itself.
For the people who don’t know what I am referring to is, All the database-related operations will be part of the model file itself and help to make a separation between your controlling logic and database logic.
For example,
Fetching the records
Fetching only non-deleted records
Hash your password/protected key before saving it into the database
Creating dependable entries in other collections (Much used for No-SQL)
Now you have a basic idea of why this approach is important, Let’s move to the implementation of it
Implementation
For this article, I will be assuming you already have a basic understanding of mongoose and its methods
So Let’s get started
Model file
I have a Blog model file that consists of only a few fields. Here is the model file for that
Now we have this simple method, I want to create a method as such that will only return me non deleted fields. To make it work let’s head back to the model file and create a new static method named findNonDeleted . This method will return all the entries from the collection whose
// syntax
Schema.static.methodName = function (...)
{
isDeleted: false
}
Adding a new method
To create a method we need to use static property from schema like this
Do not use arrow method as arrow method won’t bind your this keyword
To use this method, we can follow the file command
a script that will first delete all the blogs and then create 2 more blogs, one of them is deleted and see if the output is the same or not. Following will return the output of 1 and 2 respectively
What if you don’t want a static method but an object
There might be the change you don’t want to use class method but object method and cases can be like
Compare the password
Test if some condition is met of a specific record
Or similar
To create an object method you can follow the syntax
Schema.methods.methodName= function(parameters) { ... }
To test this you can add comparePassword the method in the Auth model like
compare the password method on the model which will decrypt the password and see if the current password is the same as the existing one
How to use object method
To use the object method, you need model instance and attach the method to it like following
It is really simple to use and create these methods but there might be some cases where you want to use pre and post callback of any method such as save, find or findOne
To see how these works follow the next heading
How to use pre and post on the schema
the syntax for the following is
Schema.pre('save', function(next) { ... }
You can use postin the same manner as pre and can replace save with any of the existing methods
Here is the example of how to use,
pre-event of save method to the user to generate a hash of the password and store it in the database
Conclusion
There are tons of use cases that you can cover with the knowledge you just received and make your project one of the best in town.
Do not use arrow method as arrow method won’t bind your this keyword
If you like my content, do consider subscribing to my substack account as well here
Hope you have leant something new and Thanks